在 Go 中使用 Electron API 创建 GUI
本篇文章介绍如何在 Go 语言中使用 Electron API 创建 GUI。
在 Go 中使用 Electron API 创建 GUI
Electron API 或 Astilectron 用于为 GoLang 创建 GUI; Astilectron 是由 Electron 驱动的封装。 这个 API 在 GitHub 上提供,它是 Electron API 的一个版本,可以使用 HTML、CSS、Javascript 和 GoLang 生成应用程序。
在使用 Electron 包之前,我们需要从 GitHub 上获取它。 让我们尝试获取并运行 Electron 包的官方演示。
获取 Electron 包并运行演示
按照以下步骤安装并运行 Astilectron 演示:
-
第一步是导入 Astilectron。 在你的项目 src 目录下的 cmd 中运行以下命令:
$ go get -u github.com/asticode/go-astilectron
-
下载 go-astilectron 后,下一步就是获取演示。 运行以下命令获取演示:
$ go get -u github.com/asticode/go-astilectron-demo/...
- 下载 Electron 后,下一步是从 YourProject/src/github.com/asticode/go-astilectron-demo/ 目录中删除 bind.go 文件。 删除该文件,以便我们稍后绑定它。
-
下一步是安装 astilectron 捆绑器。 运行以下命令下载并安装捆绑器:
$ go get -u github.com/asticode/go-astilectron-bundler/... $ go install github.com/asticode/go-astilectron-bundler/astilectron-bundler
- 现在,转到 C:\Users\Sheeraz\go\src\github.com\asticcode\go-astilectron-demo 目录并运行以下命令: ```bash #go to the directory cd C:\Users\Sheeraz\go\src\github.com\asticode\go-astilectron-demo
astilectron-bundler command
astilectron-bundler
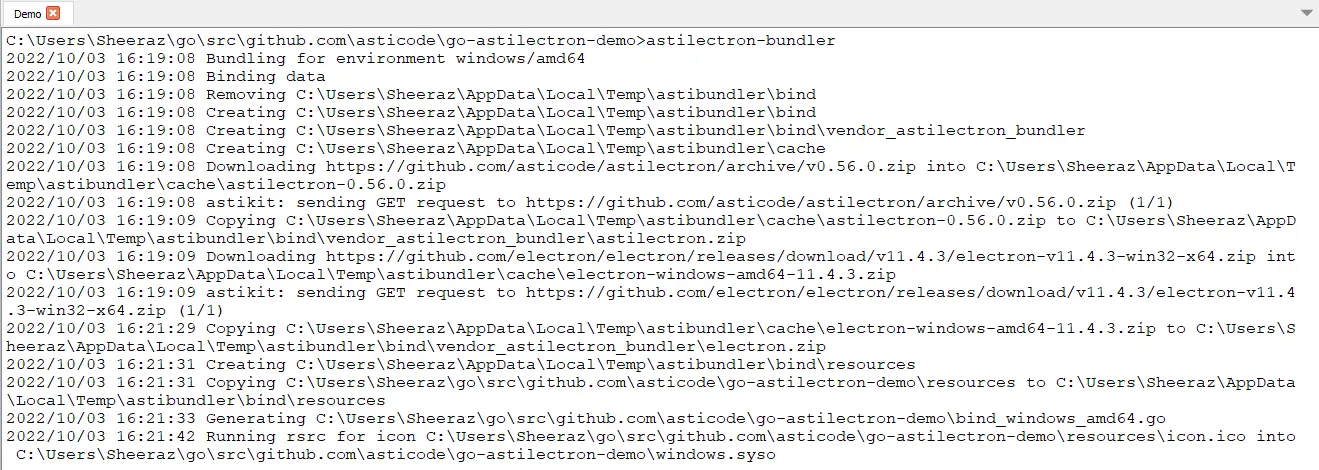
* 一旦 Bundler 命令成功,我们就可以测试演示了。 进入 C:\Users\Sheeraz\go\src\github.com\asticcode\go-astilectron-demo\output\windows-amd64 目录,运行 Astilectron demo.exe 文件:
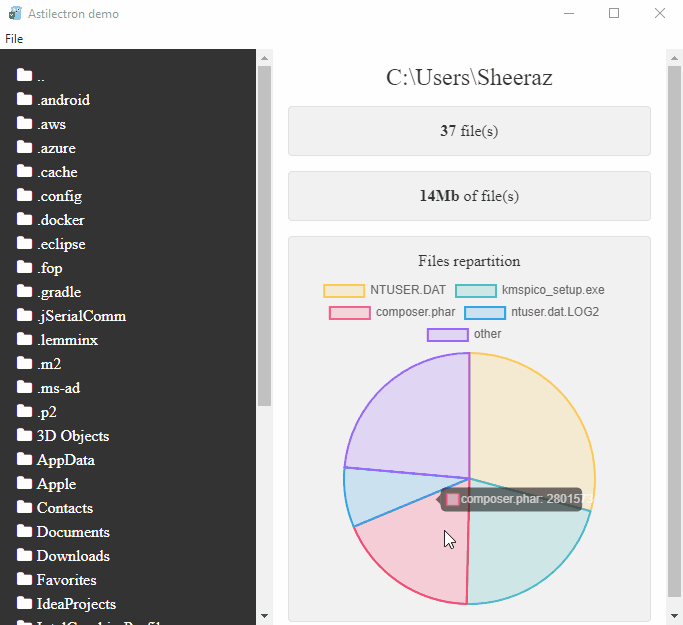
* 截至目前,我们可以看到 Astilectron 在 Windows 上的演示,它显示了打开文件夹的内存结构,但我们也可以捆绑其他操作系统的演示。 将以下部分添加到 go-astilectron-demo 目录中的 bundler.json 文件中:
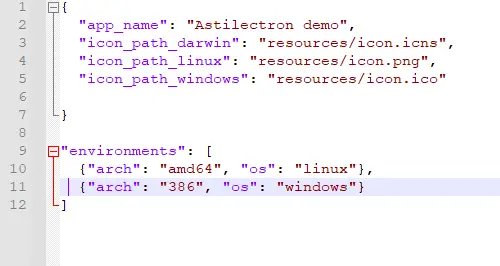
* 添加环境后,我们必须重复该过程,为所需的操作系统创建新的 Astilectron demo.exe 文件。
***
## 使用 Astilectron 的 HTML 示例
在安装 Astilectron 的上述步骤之后,我们现在可以为应用程序创建我们自己的 GUI。 让我们尝试一个使用 HTML 和 CSS 的基本示例:
用于构建和运行 HTML 应用程序的 GoLang 代码:
```go
package main
import (
"fmt"
"log"
"github.com/asticode/go-astikit"
"github.com/asticode/go-astilectron"
)
func main() {
// Set the logger
demologger := log.New(log.Writer(), log.Prefix(), log.Flags())
// Create astilectron with the application name and base directory
demoastilectron, apperror := astilectron.New(demologger, astilectron.Options{
AppName: "Jiyik",
BaseDirectoryPath: "Demo",
})
if apperror != nil {
demologger.Fatal(fmt.Errorf("main: creating the astilectron is failed: %w", apperror))
}
defer demoastilectron.Close()
// Handle the signals
demoastilectron.HandleSignals()
// Start Creating the app
if apperror = demoastilectron.Start(); apperror != nil {
demologger.Fatal(fmt.Errorf("main: starting the astilectron is failed: %w", apperror))
}
// Create a New Window
var win *astilectron.Window
if win, apperror = demoastilectron.NewWindow("index.html", &astilectron.WindowOptions{
Center: astikit.BoolPtr(true),
Height: astikit.IntPtr(800),
Width: astikit.IntPtr(800),
}); apperror != nil {
demologger.Fatal(fmt.Errorf("main: new window creation is failed: %w", apperror))
}
if apperror = win.Create(); apperror != nil {
demologger.Fatal(fmt.Errorf("main: new window creation is failed: %w", apperror))
}
// Blocking pattern
demoastilectron.Wait()
}
上面的代码还使用了 github.com/asticcode/go-astikit,它会在运行应用程序时由 IDE 下载。 HTML 的代码是:
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Jiyik Login Form</title>
<style>
body {
background-color: lightgreen;
font-size: 1.6rem;
font-family: "Open Sans", sans-serif;
color: #2b3e51;
}
h2 {
font-weight: 300;
text-align: center;
}
p {
position: relative;
}
input {
display: block;
box-sizing: border-box;
width: 100%;
outline: none;
height: 60px;
line-height: 60px;
border-radius: 4px;
}
input[type="text"],
input[type="email"] {
width: 100%;
padding: 0 0 0 10px;
margin: 0;
color: #8a8b8e;
border: 1px solid #c2c0ca;
font-style: normal;
font-size: 16px;
position: relative;
display: inline-block;
background: none;
}
input[type="submit"] {
border: none;
display: block;
background-color: lightblue;
color: #fff;
font-weight: bold;
text-transform: uppercase;
font-size: 18px;
text-align: center;
}
#login-form {
background-color: #fff;
width: 35%;
margin: 30px auto;
text-align: center;
padding: 20px 0 0 0;
border-radius: 4px;
box-shadow: 0px 30px 50px 0px rgba(0, 0, 0, 0.2);
}
#create-account {
background-color: #eeedf1;
color: #8a8b8e;
font-size: 14px;
width: 100%;
padding: 10px 0;
border-radius: 0 0 4px 4px;
}
</style>
</head>
<body>
<div id="login-form">
<h2>Jiyik.com Login</h2>
<form >
<p>
<input type="text" id="username" name="username" placeholder="Username" required><i class="validation"><span></span><span></span></i>
</p>
<p>
<input type="email" id="email" name="email" placeholder="Email Address" required><i class="validation"><span></span><span></span></i>
</p>
<p>
<input type="submit" id="login" value="Login">
</p>
</form>
<div id="create-account">
<p>Don't have an account? <a href="#">Register Here</a><p>
</div>
</div>
</body>
</html>
上面的 Go 代码将在 go-astilectron 窗口中运行给定的 HTML 代码。
相关文章
在 Golang 中使用 If-Else 和 Switch Loop Inside HTML 模板
发布时间:2023/04/27 浏览次数:101 分类:Go
-
本篇文章介绍了在 Golang 的 HTML 模板中使用 if-else 和 switch 循环。因此,只要输出是 HTML,就应该始终使用 HTML 模板包而不是文本模板。
Golang 中的零值 Nil
发布时间:2023/04/27 浏览次数:184 分类:Go
-
本篇文章介绍 nil 在 Golang 中的含义,nil 是 Go 编程语言中的零值,是众所周知且重要的预定义标识符。
Golang 中的 Lambda 表达式
发布时间:2023/04/27 浏览次数:691 分类:Go
-
本篇文章介绍如何在 Golang 中创建 lambda 表达式。Lambda 表达式似乎不存在于 Golang 中。 函数文字、lambda 函数或闭包是匿名函数的另一个名称。
在 Go 中捕获 Panics
发布时间:2023/04/27 浏览次数:104 分类:Go
-
像错误一样,Panic 发生在运行时。 换句话说,当您的 Go 程序中出现意外情况导致执行终止时,就会发生 Panics。让我们看一些例子来捕捉 Golang 中的Panics。
在 Go 中使用断言
发布时间:2023/04/27 浏览次数:585 分类:Go
-
本篇文章介绍了 assert 在 GoLang 中的使用。在 Go 语言中使用断言:GoLang 不提供对断言的任何内置支持,但我们可以使用来自 Testify API 的广泛使用的第三方包断言。
Go 中的随机数生成
发布时间:2023/04/27 浏览次数:206 分类:Go
-
本篇文章介绍如何在 Go 语言中使用随机数生成功能。Go 中的随机数生成 Go 语言为随机数生成功能提供内置支持。 内置包 math 有方法 rand(),用于随机数生成。